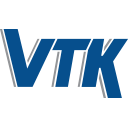 |
VTK
9.4.20250221
|
Go to the documentation of this file.
5#ifndef vtkDeprecation_h
6#define vtkDeprecation_h
8#include "vtkVersionQuick.h"
46#ifndef VTK_DEPRECATION_LEVEL
48#ifdef VTK_VERSION_NUMBER
49#define VTK_DEPRECATION_LEVEL VTK_VERSION_NUMBER
51#define VTK_DEPRECATION_LEVEL VTK_VERSION_NUMBER_QUICK
56#define VTK_MINIMUM_DEPRECATION_LEVEL VTK_VERSION_CHECK(9, 3, 0)
60#if VTK_DEPRECATION_LEVEL < VTK_MINIMUM_DEPRECATION_LEVEL
61#undef VTK_DEPRECATION_LEVEL
62#define VTK_DEPRECATION_LEVEL VTK_MINIMUM_DEPRECATION_LEVEL
66#if 0 && __cplusplus >= 201402L
69#define VTK_DEPRECATION(reason) [[deprecated(reason)]]
70#elif defined(VTK_WRAPPING_CXX)
72#define VTK_DEPRECATION(reason)
73#elif defined(__VTK_WRAP__)
74#define VTK_DEPRECATION(reason) [[vtk::deprecated(reason)]]
76#if defined(_WIN32) || defined(_WIN64)
77#define VTK_DEPRECATION(reason) __declspec(deprecated(reason))
78#elif defined(__clang__)
79#if __has_extension(attribute_deprecated_with_message)
80#define VTK_DEPRECATION(reason) __attribute__((__deprecated__(reason)))
82#define VTK_DEPRECATION(reason) __attribute__((__deprecated__))
84#elif defined(__GNUC__)
85#if (__GNUC__ >= 5) || ((__GNUC__ == 4) && (__GNUC_MINOR__ >= 5))
86#define VTK_DEPRECATION(reason) __attribute__((__deprecated__(reason)))
88#define VTK_DEPRECATION(reason) __attribute__((__deprecated__))
91#define VTK_DEPRECATION(reason)
96#if defined(__VTK_WRAP__)
97#define VTK_DEPRECATED_IN_9_5_0(reason) [[vtk::deprecated(reason, "9.5.0")]]
98#elif VTK_DEPRECATION_LEVEL >= VTK_VERSION_CHECK(9, 4, 20241008)
99#define VTK_DEPRECATED_IN_9_5_0(reason) VTK_DEPRECATION(reason)
101#define VTK_DEPRECATED_IN_9_5_0(reason)
105#if defined(__VTK_WRAP__)
106#define VTK_DEPRECATED_IN_9_4_0(reason) [[vtk::deprecated(reason, "9.4.0")]]
107#elif VTK_DEPRECATION_LEVEL >= VTK_VERSION_CHECK(9, 3, 20230807)
108#define VTK_DEPRECATED_IN_9_4_0(reason) VTK_DEPRECATION(reason)
110#define VTK_DEPRECATED_IN_9_4_0(reason)
114#if defined(__VTK_WRAP__)
115#define VTK_DEPRECATED_IN_9_3_0(reason) [[vtk::deprecated(reason, "9.3.0")]]
117#define VTK_DEPRECATED_IN_9_3_0(reason) VTK_DEPRECATION(reason)
120#if defined(__VTK_WRAP__)
121#define VTK_DEPRECATED_IN_9_2_0(reason) [[vtk::deprecated(reason, "9.2.0")]]
123#define VTK_DEPRECATED_IN_9_2_0(reason) VTK_DEPRECATION(reason)
126#if defined(__VTK_WRAP__)
127#define VTK_DEPRECATED_IN_9_1_0(reason) [[vtk::deprecated(reason, "9.1.0")]]
129#define VTK_DEPRECATED_IN_9_1_0(reason) VTK_DEPRECATION(reason)
132#if defined(__VTK_WRAP__)
133#define VTK_DEPRECATED_IN_9_0_0(reason) [[vtk::deprecated(reason, "9.0.0")]]
135#define VTK_DEPRECATED_IN_9_0_0(reason) VTK_DEPRECATION(reason)
138#if defined(__VTK_WRAP__)
139#define VTK_DEPRECATED_IN_8_2_0(reason) [[vtk::deprecated(reason, "8.2.0")]]
141#define VTK_DEPRECATED_IN_8_2_0(reason) VTK_DEPRECATION(reason)